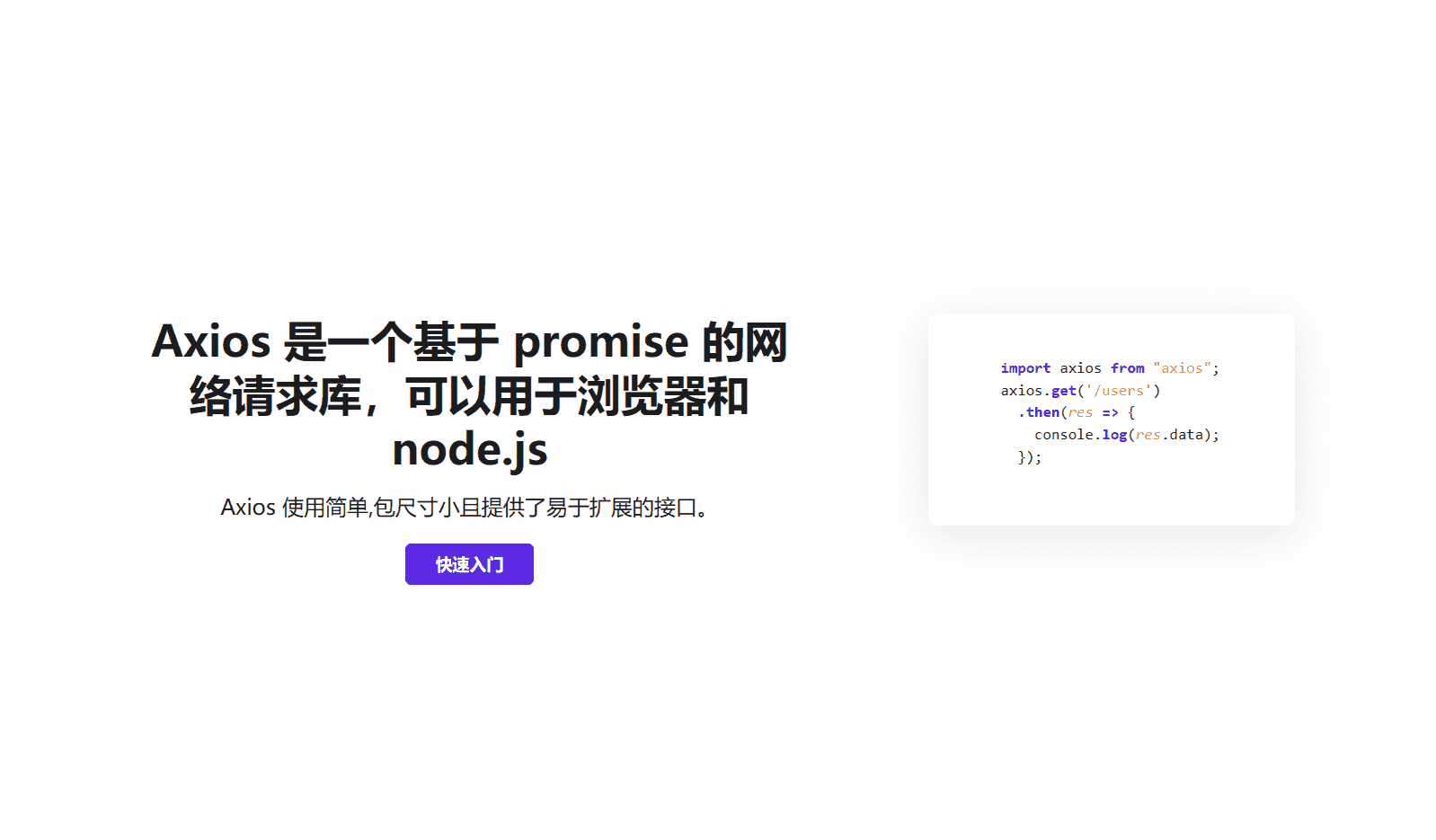
Axios请求库实战
AI-摘要
切换
小郭 GPT
AI初始化中...
介绍自己
生成本文简介
推荐相关文章
前往主页
前往tianli博客
本文最后更新于 2024-03-12,文章发布日期超过365天,内容可能已经过时。
🦝axios教程
一、axios介绍
axios是基于promise对ajax的一种封装
ajax mvc
axios mvvm
二、axios的基本使用
发送get请求
//使用默认方式发送无参请求
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios({
url:'http://localhost:9999/student/student/getAllStudent'
}).then(res=>{
console.log(res);
})
</script>
//默认使用 get方式进行请求
//指定请求方式为get的无参请求
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios({
url:'http://localhost:9999/student/student/getAllStudent',
method:'get'
}).then(res=>{
console.log(res);
})
</script>
//axios发送get方式的有参请求
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios({
url:'http://localhost:9999/student/student/findStudentById?id=1'
}).then(res=>{
console.log(res);
});
</script>
//axios发送get方式请求其他形式
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios({
url:'http://localhost:9999/student/student/findStudentById',
params:{
id:'1'
}
}).then(res=>{
console.log(res);
});
</script>
发送post请求
//使用post方式发送无参请求
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios({
url:'http://localhost:9999/student/student/pGet',
method:'post'
}).then(res=>{
console.log(res);
})
</script>
//使用axios发送带有参数的post请求
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios({
url:'http://localhost:9999/student/student/findStudentByName',
method:'post',
params:{
name:'张三' //url上拼接上name参数 ?name=张三
}
}).then(res=>{
console.log(res);
});
</script>
//使用axios发送带有参数的post请求 使用data传递
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios({
url:'http://localhost:9999/student/student/findStudentByName',
method:'post',
data:{
name:'张三' //后台接收为null
}
}).then(res=>{
console.log(res);
});
</script>
后台控制器接收到的name null,原因是axios使用post携带参数请求默认使用application/json
解决方式一: params属性进行数据的传递
解决方式二: "name=张三"
解决方式三: 服务器端给接收的参数加上@requestBody
三、axios请求简写
//使用axios.get方式发送无参请求
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios.get('http://localhost:9999/student/student/getAllStudent').then(res=>{
console.log(res);
}).catch(err=>{
console.log(err);
})
</script>
//使用axios.get发送有参请求
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios.get('http://localhost:9999/student/student/findStudentById',{params:
{id:1}}).then(res=>{
console.log(res);
}).catch(err=>{
console.log(err);
})
</script>
//使用axios.post无参请求
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios.post('http://localhost:9999/student/student/pGet').then(res=>{
console.log(res);
}).catch(err=>{
console.log(err);
})
</script>
//使用axios.post发送有参请求 只修改前端代码
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios.post('http://localhost:9999/student/student/findStudentByName',"name=张三
&age=10").then(res=>{
console.log(res);
});
</script>
//发送post请求携带参数 直接试用"name=张三&age=10"
//使用data传递数据 后台需要将axiso自动装换的json数据装换为java对象 //修改后台代码
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios.post('http://localhost:9999/student/student/findStudentByName',{name:'张三'})
.then(res=>{
console.log(res);
});
</script>
//接收参数添加@requestBody注解
四、axios的并发请求
//axios的并发请求
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios.all([
axios.get('http://localhost:9999/student/student/getAllStudent'),
axios.get('http://localhost:9999/student/student/findStudentById',{params:{id:1}})
]).then(res=>{//请求成功响应的是一个数组
console.log(res[0]);
console.log("----------");
console.log(res[1]);
}).catch(err=>{
console.log(err);
})
</script>
//使用spread方法处理响应数组结果
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios.all([
axios.get('http://localhost:9999/student/student/getAllStudent'),
axios.get('http://localhost:9999/student/student/findStudentById',{params:{id:1}})
]).then(
axios.spread((res1,res2)=>{
console.log(res1);
console.log(res2);
})
).catch(err=>{
console.log(err);
})
</script>
五、axios的全局配置
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios.defaults.baseURL='http://localhost:9999/student/student';//配置全局属性
axios.defaults.timeout=5;
axios.get('getAllStudent').then(res=>{ //在全局配置的基础上去网络请求
console.log(res);
});
axios.post('pGet').then(res=>{
console.log(res);
}).catch(err=>{
console.log(err);
})
</script>
六、axios的实例
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
let newVar = axios.create({
baseURL:'http://localhost:9999/student/student',
timeout:5000
});//创建axios的实例
let newVar1 = axios.create({
baseURL:'http://localhost:9999/student',
timeout:5000
});//创建axios的实例
newVar({
url:'getAllStudent'
}).then(res=>{
console.log(res);
})
newVar1({
url:'student/getAllStudent'
}).then(res=>{
console.log(res);
}).catch(err=>{
console.log(err);
})
</script>
七、axios的拦截器
axios给我们提供了两大类拦截器 一种是请求方向的拦截(成功请求,失败的)
另一种是响应方向的(成功的 失败的)
拦截器的作用 用于我们在网络请求的时候在发起请求或者响应时对操作进行响应的处理
发起请求时可以添加网页加载的动画 强制登录
响应的时候可以进行相应的数据处理
//请求方向拦截器
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios.interceptors.request.use(config=>{
console.log("进入请求拦截器");
console.log(config);
return config;//放行请求
},err=>{
console.log("请求方向失败");
console.log(err);
});
axios.get("http://localhost:9999/student/student/getAllStudent").then(res=>{
console.log(res);
})
</script>
//响应方向拦截器
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
axios.interceptors.response.use(config=>{
console.log("进入响应拦截器");
return config.data;
},err=>{
console.log("响应方向失败");
console.log(err);
});
axios.get("http://localhost:9999/student/student/getAllStudent").then(res=>{
console.log(res);
})
</script>
八、axios在vue中的模块封装
//封装位置 文件绝对位置./network/request/request.js
import axios from 'axios'
export function request(config,success,fail) {
axios({
url:config
}).then(res=>{
success(res);
}).catch(err=>{
fail(err);
})
}
//调用者位置
import {request} from './network/request/request'
request('http://localhost:9999/student/student/getAllStudent',res=>{
console.log(res);
},err=>{
console.log(err);
})
//封装位置
export function request(config){
axios.defaults.baseURL="http://localhost:9999/student/student";
axios(config.url
).then(res=>{
config.success(res);
}).catch(err=>{
config.fail(err);
})
}
//调用者位置
import {request} from './network/request/request'
request({
url:'getAllStudent',
success:res=>{
console.log(res);
},
fail:err=>{
console.log(err);
}
})
//封装的位置
export function request(config){
return new Promise((resolve, reject) => {
let newVar = axios.create({
baseURL:"http://localhost:9999/student/student",
timeout:5000
});
newVar(config).then(res=>{
resolve(res);
}).catch(err=>{
reject(err);
})
})
}
//调用位置
import {request} from './network/request/request'
request({
url:'getAllStudent'
}).then(res=>{
console.log(res);
}).catch(err=>{
console.log(err);
})
//封装位置
export function request(config){
let newVar = axios.create({
baseURL:"http://localhost:9999/student/student",
timeout:5000
});
return newVar(config);
//调用者
import {request} from './network/request/request'
request({
url:'getAllStudent'
}).then(res=>{
console.log(res);
})
🐻axios实战
login.js
// 定义登录和注册的工具函数
// 导入Axios库
import axios from "axios";
const baseURL = import.meta.env.VITE_BASE_URL + "/login";
const timeout = 10000;
var headers = {
"Access-Control-Allow-Origin": "*",
"Content-Type": "application/json",
};
// 创建 Axios 实例
var request = axios.create({
baseURL: baseURL,
timeout: timeout,
headers: headers,
});
const login = async (requestData) => {
let res = await request.post("", requestData);
return res;
};
const register = async (requestData) => {
let res = await request.put("", requestData);
return res;
};
// 导出函数
export { login, register };
request.js
// 定义需要带token请求的工具函数
// 导入 Axios 库
import axios from "axios";
import router from "@/router";
const baseURL = import.meta.env.VITE_BASE_URL;
const timeout = 10000;
const initRequest = () => {
const headers = {
"Access-Control-Allow-Origin": "*",
Token: localStorage.getItem("Token"),
"Content-Type": "application/json",
};
// 创建 Axios 实例
const request = axios.create({
baseURL: baseURL,
timeout: timeout,
headers: headers,
});
return request;
};
// 判断登录是否过期
const generalCheck = (responseData) => {
// 如果返回 true 则不需要处理
if (responseData.data.code == 401) {
// 登录过期或没有登录,清除 Token 并跳转到登录页面
localStorage.removeItem("Token");
router.push("/login");
return true;
}
return false;
};
// 定义需要带 Token 请求的工具函数
const get = (url, requestData, success, fail) => {
initRequest()
.get(url, { data: requestData })
.then((res) => {
if (res.data.code === 200) {
// 只有响应码 200 的情况才是正确的
success(res);
} else {
if (!generalCheck(res)) fail(res);
}
})
.catch((err) => {
// 发请求失败,跳转到 404 页面
router.push("/404");
});
};
const put = (url, requestData, success, fail) => {
// 使用 put 传递请求体,直接传递 requestData 即可
initRequest()
.put(url, requestData)
.then((res) => {
if (res.data.code === 200) {
success(res);
} else {
if (!generalCheck(res)) fail(res);
}
})
.catch((err) => {
router.push("/404");
});
};
const post = (url, requestData, success, fail) => {
// 使用 post 传请求体,只有直接写 requestData 才能传递请求体
initRequest()
.post(url, requestData)
.then((res) => {
if (res.data.code === 200) {
success(res);
} else {
if (!generalCheck(res)) fail(res);
}
})
.catch((err) => {
router.push("/404");
console.log("post请求失败,then都没进去");
});
};
const del = (url, requestData, success, fail) => {
// 使用 delete 传请求体,参数格式为 {data: requestData}、{params: requestData}
initRequest()
.delete(url, { data: requestData })
.then((res) => {
if (res.data.code === 200) {
success(res);
} else {
if (!generalCheck(res)) fail(res);
}
})
.catch((err) => {
router.push("/404");
});
};
const patch = (url, requestData, success, fail) => {
// 使用 patch 传请求体,只有直接写 requestData
initRequest()
.patch(url, requestData)
.then((res) => {
if (res.data.code === 200) {
success(res);
} else {
if (!generalCheck(res)) fail(res);
}
})
.catch((err) => {
router.push("/404");
});
};
// 导出函数
export { get, put, post, del, patch };
使用举例
// 从后端获取requestData
// 编辑请求信息
const requestData = ref({
"username": user.value.username
})
// 发送请求
post(url, requestData.value,
res => {
responseData.value = res.data // 将响应数据存储在响应式变量中
console.log('获取比赛列表数据成功')
console.log(res.data)
},
err => {
console.error(err)
console.log('获取比赛列表数据失败')
}
)
// 面板基本信息修改按钮点击事件
const trueEditBasic = () => {
put('/game', {
"user": {
"username": userName.value
},
"game": {
"id": basicForm.value.id,
"gameName": basicForm.value.gameName,
"timeStart": timeTransform(basicForm.value.timeStart1, basicForm.value.timeStart2),
"timeEnd": timeTransform(basicForm.value.timeEnd1, basicForm.value.timeEnd2),
"gameIntro": basicForm.value.gameIntro
}
},
res => {
ElMessage({
type: 'success',
message: '修改成功',
})
// 更新比赛列表的数据
post('/games', {
"username": userName.value
},
res => {
adminResponseData.value = res.data // 将响应数据存储在响应式变量中
console.log('更新比赛列表数据成功')
console.log(res.data)
},
err => {
console.error(err)
console.log('更新比赛列表数据失败')
}
)
},
err => {
console.error(err.data)
ElMessage({
type: 'error',
message: '修改失败',
})
}
)
}
- 感谢你赐予我前进的力量
赞赏者名单
因为你们的支持让我意识到写文章的价值🙏
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 郭同学的笔记本
评论
匿名评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果